I separated out the relevant parts of your .ino file for examination and discussion here ...
const int MPU_addr1 = 0x68;
float xa, ya, za, roll, pitch, gyroX, gyroY;
...
roll = atan2(ya , za) * 180.0 / PI;
pitch = atan2(-xa , sqrt(ya * ya + za * za)) * 180.0 / PI;
gyroX = pitch;
gyroY = roll ;
Serial.print("sumbu X = ");
Serial.print(gyroX,1);
Serial.println(;);
Serial.print(", sumbu Y = ");
Serial.println(gyroY,1);
...
// Perintah untuk menggerakkan aktuator (bluetooth)
while(Serial.available()>0)
{
char value = Serial.read(); // reading the data received from the bluetooth module
Serial.println (value);
if (value == '1'){
digitalWrite(X1, LOW);
digitalWrite(X2, HIGH);;
}
if (value == '2'){
digitalWrite(X1, HIGH);
digitalWrite(X2, LOW);
}
...
I notice a few problematic things in your print stream to Serial, which I imagine is your BlueTooth stream going to AI2 ...
- You println between gyroX and gyroY, forcing them into separate lines, but you also include a comma between their values. This complicates parsing in AI2, which I will examine further down.
- You include commentary (name = ...) in your print stream but you do not separate that out in Ai2. (This has all the symptoms of the two programs being written by two different people who don't talk to each other.)
- You echo back single chaarcter BlueTooth commands back to AI2. That complicates parsing the BlueTooth input stream in AI2.
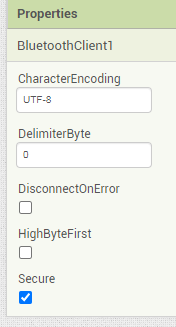
Your BlueTooth Delimiter Byte is not set to 10 (Line Feed) in the Designer.
Let's see if it is set in your blocks ...
No, you don't. That is an error.
Let's look at your blank block you use for splitting.
I used the length() block to test a copy of it.
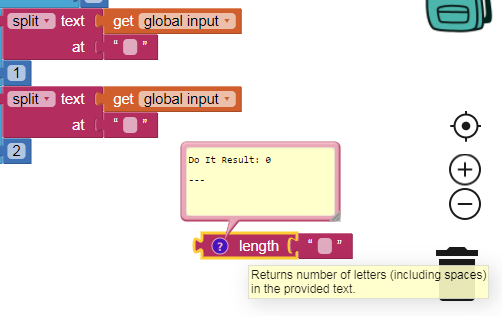
Surprise!
A zero length. A blank would have a length of 1.
Here is a present for you, a draggable block you can pull into your Blocks Editor across browser windows ...

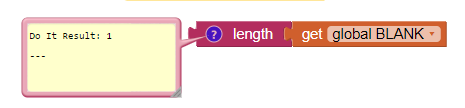
Since all your errors come from not doing standard delimiter handling, I will give you the stock standard BlueTooth Delimiter advice now.
Please see the Delimiter article in FAQ
Be sure to use println() at the end of each message to send from the sending device, to signal end of message. Do not rely on timing for this, which is unreliable.
In the AI2 Designer, set the Delimiter attribute of the BlueTooth Client component to 10 to recognize the End of Line character.
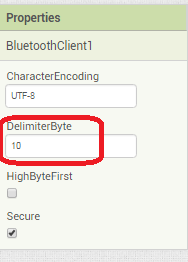
Also, return data is not immediately available after sending a request,
you have to start a Clock Timer repeating and watch for its arrival in the Clock Timer event. The repeat rate of the Clock Timer should be faster than the transmission rate in the sending device, to not flood the AI2 buffers.
In your Clock Timer, you should check
Is the BlueTooth Client still Connected?
Is Bytes Available > 0?
IF Bytes Available > 0 THEN
set message var to BT.ReceiveText(-1)
This takes advantage of a special case in the ReceiveText block:
ReceiveText(numberOfBytes)
Receive text from the connected Bluetooth device. If numberOfBytes is less than 0, read until a delimiter byte value is received.
If you are sending multiple data values per message separated by | or comma, have your message split into a local or global variable for inspection before trying to select list items from it. Test if (length of list(split list result) >= expected list length) before doing any select list item operations, to avoid taking a long walk on a short pier. This bulletproofing is necessary in case your sending device sneaks in some commentary messages with the data values.